1. Serializable, ObjectOutputStream, FileOutputStream
import java.io.FileOutputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
import java.util.*;
class Sawon implements Serializable
{
private int sabun;
private String name;
private String dept;
public int getSabun() {
return sabun;
}
public void setSabun(int sabun) {
this.sabun = sabun;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDept() {
return dept;
}
public void setDept(String dept) {
this.dept = dept;
}
}
public class M1 {
public static void main(String[] args) {
Sawon sa = new Sawon();
sa.setSabun(1);
sa.setName("김");
sa.setDept("개발부");
try
{
// ArrayList 자체 저장 -> stringTokenizer, split 사용 필요X
FileOutputStream fos = new FileOutputStream("c:\\사원\\object.txt"); // 파일 쓰기
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(sa);
oos.close();
fos.close();
System.out.println("객체 단위 저장 완료!!");
} catch(Exception ex) {}
}
}
깨져서 나옴
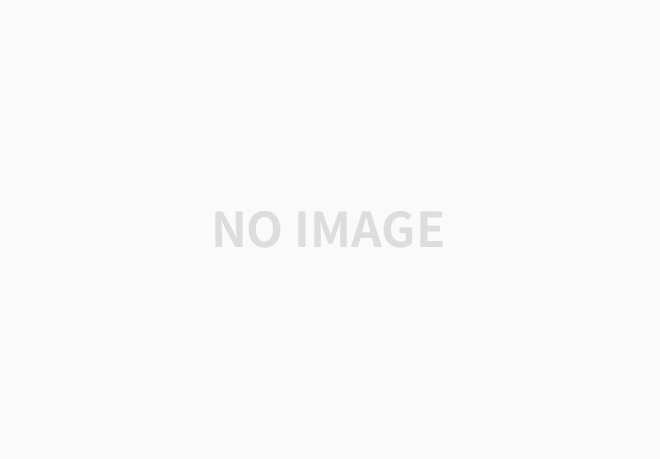
2.
import java.io.FileInputStream;
import java.io.ObjectInputStream;
public class M2 {
public static void main(String[] args) {
try
{
FileInputStream fis = new FileInputStream("c:\\사원\\object.txt");
ObjectInputStream ois = new ObjectInputStream(fis);
Sawon sa = (Sawon)ois.readObject();
System.out.println(sa.getDept());
System.out.println(sa.getName());
System.out.println(sa.getSabun());
} catch(Exception ex) {}
}
}
개발부
김
1
3. 직렬화로 평균 구하기
import java.util.*;
import java.io.*;
class Student implements Serializable
{
private int hakbun;
private String name;
private int kor, eng, math;
public int getHakbun() {
return hakbun;
}
public void setHakbun(int hakbun) {
this.hakbun = hakbun;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getKor() {
return kor;
}
public void setKor(int kor) {
this.kor = kor;
}
public int getEng() {
return eng;
}
public void setEng(int eng) {
this.eng = eng;
}
public int getMath() {
return math;
}
public void setMath(int math) {
this.math = math;
}
// 매개변수 있는 생성자
public Student(int hakbun, String name, int kor, int eng, int math) {
this.hakbun = hakbun;
this.name = name;
this.kor = kor;
this.eng = eng;
this.math = math;
}
}
public class M3 {
public static void main(String[] args) {
ArrayList<Student> list = new ArrayList<Student>();
list.add(new Student(1, "김", 80, 90, 70));
list.add(new Student(2, "이", 82, 92, 72));
list.add(new Student(3, "박", 83, 93, 73));
list.add(new Student(4, "최", 84, 94, 74));
list.add(new Student(5, "유", 85, 95, 75));
// 저장
try
{
FileOutputStream fos = new FileOutputStream("c:\\사원\\student.txt");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(list);
oos.close();
fos.close();
System.out.println("저장 완료!!");
} catch(Exception ex) {}
}
}
import java.util.*;
import java.io.*;
public class M4 {
public static void main(String[] args) {
try
{
FileInputStream fis = new FileInputStream("c:\\사원\\student.txt");
ObjectInputStream ois = new ObjectInputStream(fis);
ArrayList<Student> list = (ArrayList<Student>)ois.readObject();
for(Student s:list)
{
System.out.println
(
s.getHakbun() + " "
+ s.getName() + " "
+ s.getKor() + " "
+ s.getEng() + " "
+ s.getMath() + " "
+ (s.getKor() + s.getEng() + s.getMath()) + " "
+ String.format("%.2f", (s.getKor() + s.getEng() + s.getMath())/3.0));
}
} catch(Exception ex) {}
}
}
1 김 80 90 70 240 80.00
2 이 82 92 72 246 82.00
3 박 83 93 73 249 83.00
4 최 84 94 74 252 84.00
5 유 85 95 75 255 85.00
4. IO 응용
'수업' 카테고리의 다른 글
+29 라이브러리(IO) : 스트림, 직렬화(ObjectInputStream, ObjectOutputStream) (0) | 2022.06.14 |
---|---|
+28 라이브러리(ArrayList), Stack, Queue, HashSet, TreeSet, HashMap, Collections, 어노테이션 (0) | 2022.06.13 |
+27 컬렉션 프레임워크: ArrayList (0) | 2022.06.10 |
+26 형식화 클래스: Decimal Format, SimpleDateFormat, ChoiceFormat (0) | 2022.06.09 |
+26 라이브러리 : java.util, java.util.regex, java.util.StringTokenizer (0) | 2022.06.09 |